In the previous post, we’ve learned how to build a simple single-page application (SPA) using React Router. In this article, we will learn to animate route transitions using React Router Transition package.
Prerequisites
We expect that you are familiar with the following:
- JavaScript (Basic level)
- CSS (Basic level)
- ReactJs (Basic level, must understand What is Component & React Router)
Expected Learnings
Your key learnings from this project will be:
- A basic example of adding animated transition to React Router.
Step 1: Install React Router Transition
To get started, you need to have a ready installed single page application (SPA), if you haven’t already, be sure to read the Beginner’s Guide to React Router first!
Once you have installed a single page application (SPA) on your computer, the next thing is to install the React Router Transition by running following command:
npm install --save react-router-transition
React Router Transition is a simple package that offers you to add painless transition to your single page application. It was really easy to learn and adapt it. If your app doesn’t requires much complex transitions, this package definitely suit your need.
Once the installation is complete, import the React Router Transition object into the App.js. Once that is done, we can start to add animated transition to the Route.
import { AnimatedSwitch } from 'react-router-transition';
Step 2: Setting Up Transition
Refactored <Switch> to <AnimatedSwitch> for setting up the transition.
<AnimatedSwitch> just like <Switch> , but with transitions when the child route changes. Allows for animating sibling routes with a mounting and unmounting transition.
The <AnimatedSwitch> offers several props:
- atEnter, atLeave, atActive
- Objects with numerical values, expressing the interpolatable states a component will have when mounting, unmounting and mounted, respectively. Note that spring objects are valid only for atActive and atLeave.
- mapStyles
- An optional function for transforming values that don’t map 1:1 with styles (e.g. translateX or other values of the transform style property).
- runOnMount
- A boolean flag to signal whether or not to apply a transition to the child component while mounting the parent.
- wrapperComponent
- The element type (‘div’, ‘span’, etc.) to wrap the transitioning routes with. Use false to transition child components themselves, though this requires consuming a style prop that gets injected into your component.
- className
- A class name to apply to the root node of the transition.
Let’s make a simple example of fading + sliding in a component on enter (atEnter) and fading + sliding it out on exit (atLeave) might look something like this:
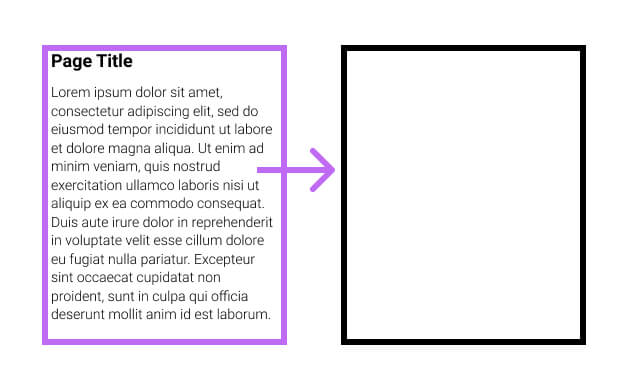
import './App.css';
import { Fragment } from 'react';
import { BrowserRouter as Router, Route, Link, Switch } from 'react-router-dom';
import { useHistory, useRouteMatch } from 'react-router';
import { AnimatedSwitch } from 'react-router-transition';
function App() {
return (
<Router>
<main>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/shop">Shop</Link></li>
<li><Link to="/shop/1">Product 1</Link></li>
</ul>
</nav>
<AnimatedSwitch
atEnter={routeAnimation.atEnter}
atLeave={routeAnimation.atLeave}
atActive={routeAnimation.atActive}
mapStyles={mapStyles}
className="switch-wrapper"
>
<Route exact path="/">
<Home />
</Route>
<Route exact path="/about">
<About />
</Route>
<Route path="/shop">
<Shop />
</Route>
</AnimatedSwitch>
</main>
</Router>
);
}
const routeAnimation = {
atEnter: {
opacity: 0,
translateX: -100
},
atLeave: {
opacity: 0,
translateX: 100
},
atActive: {
opacity: 1,
translateX: 0
}
}
const mapStyles = (styles) => {
return {
opacity: styles.opacity,
transform: `translateX(${styles.translateX}px)`,
};
}
const Home = () => (
<Fragment>
<h1>Home</h1>
<LoremText />
</Fragment>
)
const About = () => {
const hist = useHistory();
return (
<Fragment>
<h1>About</h1>
<button onClick={() => hist.goBack()}>Go Back</button>
<LoremText />
</Fragment>
)
}
const Shop = () => {
const routeMatch = useRouteMatch('/shop/:id');
const returnRouteMatch = routeMatch ? `You requested item with ID: ${routeMatch.params.id}` : 'All product';
return (
<Fragment>
<h1>Shop</h1>
<p>{`${returnRouteMatch}`}</p>
</Fragment>
)
}
const LoremText = () => (
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
</p>
)
export default App;
This illustrates <AnimatedSwitch> uses these 3 main states to complete a transition:
- atEnter: Where the transition mounting
- atActive: Where the transition mounted
- atLeave: Where the transition unmounting
Moreover, we’ve added a custom class “switch-wrapper” for CSS styles.
Next, add following CSS styles in App.css for the <nav> & <AnimatedSwitch>:
body {
margin: 0 auto;
width: 320px;
}
nav ul {
padding: 0;
}
nav ul li {
display: inline-block;
margin: 0 12px;
}
.switch-wrapper {
position: relative;
}
.switch-wrapper > div {
position: absolute;
}
Save your code, and reload the page in browser.
You should see the transition works as your expectation!
That’s it! 👏
In this article, we saw how to add simple route transitions using React Router Transition package. If you want to study further about React Router Transition package, you can do it here.
Follow onlyWebPro.com on Facebook now for latest web development tutorials & tips updates!